|
|
|
CREATE TABLE contact (
ID int(11) NOT NULL default ‘0’,
FIRSTNAME varchar(20), LASTNAME
varchar(20),
EMAIL varchar(25), PRIMARY KEY (ID)); |
II. Creating persistent java objects
The second step is to create persistent java
objects. In Java JDK, all primitive types (like
String, char and Date) can be mapped, including
classes from the Java collections framework.
There is also no need to implement special
interface for persistent classes because
Hibernate works well with the Plain Old Java
Objects programming model for these
persistent classes.
Following code sample represents a java
object structure that represents the “contact” table. Generally these domain
objects contain only getters and setters
methods.
Here is the code for Contact.java: package roseindia.tutorial.hibernate;
public class Contact {
private String firstName;
private String lastName;
private String email;
private int id;
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
} |
|
|
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
} |
III. Mapping the POJO’s Objects to the
Database table using hibernate mapping
document
Each persistent class needs to be mapped
with its configuration file. Following code
represents Hibernate mapping file
“contact.hbm.xml” for mapping of
Contact’s Objects to the Database Contact
table.
<?xml version=”1.0"?>
<!DOCTYPE hibernate-mapping PUBLIC
“-//Hibernate/Hibernate Mapping DTD 3.0//
EN”
“http://hibernate.sourceforge.net/hibernatemapping-
3.0.dtd”>
<hibernate-mapping>
<class
name=”roseindia.tutorial.hibernate.Contact”
table=”CONTACT”>
<id name=”id” type=”int” column=”ID” >
<generator class=”assigned”/>
</id>
<property name=”firstName”>
<column name=”FIRSTNAME” />
</property>
<property name=”lastName”>
<column name=”LASTNAME”/>
</property>
<property name=”email”>
<column name=”EMAIL”/>
</property>
</class>
</hibernate-mapping> |
Hibernate mapping documents are easy to
understand. The <class> element maps a
table with corresponding class. The <id>
element represents the primary key column,
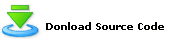
|
|
Dec
2007 | Java Jazz Up |29 |
|
|
|
View All Topics |
All Pages of this Issue |
Pages:
1,
2,
3,
4,
5,
6,
7,
8,
9,
10,
11,
12,
13,
14,
15,
16,
17,
18,
19,
20,
21,
22,
23,
24,
25,
26,
27,
28,
29,
30,
31,
32,
33,
34,
35,
36,
37,
38,
39,
40,
41,
42,
43,
44,
45,
46,
47,
48,
49,
50,
51,
52,
53 ,
54,
55,
56,
57,
58,
59,
60,
61,
62,
63 ,
64,
65 ,
66 ,
67 ,
68 ,
69 ,
70 ,
71 ,
72 ,
73 ,
74 ,
75 ,
76 ,
77 ,
78 ,
79 ,
80 ,
81 ,
82 , Download PDF |
|
|
|
|
|
|
|
|
|