|
Design Pattern |
|
TestState.java
Class TestState {
public static void main(String[] args) {
StateContext stateContext = new StateContext();
stateContext.showName(
"Mr. Deepak Kumar - "+
"IT Manager Roseindia Technologies");
stateContext.showName(
"Mr. Amit Kumar: Operational Manager
Roseindia Technologies");
stateContext.showName(
"Mr. Aquil Ahmad Khan: HR
Manager: Roseindia Technologies");
stateContext.showName(
"And this is me, Mohd. Zulfiqar Ahmed:
Senior Software Engineer
Roseindia Technologies");
}
Here is the Output:
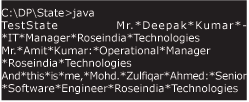
III. Strategy pattern
The Strategy pattern is a design pattern in
which an object controls which of a family of
methods is called simply. Each method of this
family stays in its own class and extends a
common base class. This method introduces a
family of algorithms, encapsulates them with
each other and makes them interchangeable.
This pattern provides pluggable behavior that
enforces the client access to services. This
pattern is used in those situations where many
related classes differs only in their behavior.
Here we are taking an example that encapsulates
several algorithms (classes) into a single module
just to provides the alternatives. These
alternatives are provided by generating random
numbers to serve the purpose. |
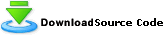 |
|
interface StrategyPattern {
public void print();
}
class GetIt implements StrategyPattern {
public void print() {
System.out.println("Still you are not late!");
}
}
class StartNow implements StrategyPattern {
public void print() {
System.out.println("You can also start from now!");
}
}
class LooseNothing implements StrategyPattern {
public void print() {
System.out.println
("You didn't loose anything so
get up and start now!");
}
}
class Dice {
public int throwIt() {
return (int)(Math.random()*6)+1;
}
}
class Test {
static void goodLuck() {
int yourluckyNum = new Dice().throwIt();
StrategyPattern sp;
switch (yourluckyNum) {
case 2: sp = new GetIt();
break;
case 5: sp = new StartNow();
break;
default: sp = new LooseNothing();
}
sp.print();
}
public static void main(String[] args) {
goodLuck();
}
}
|
Here is the output:
|
|
Feb
2008 | Java Jazz Up |61 |
|
|
View All Topics |
All Pages of this Issue |
Pages:
1,
2,
3,
4,
5,
6,
7,
8,
9,
10,
11,
12,
13,
14,
15,
16,
17,
18,
19,
20,
21,
22,
23,
24,
25,
26,
27,
28,
29,
30,
31,
32,
33,
34,
35,
36,
37,
38,
39,
40,
41,
42,
43,
44,
45,
46,
47,
48,
49,
50,
51,
52,
53 ,
54,
55,
56,
57,
58,
59,
60,
61,
62,
63 ,
64,
65 ,
66 ,
67 ,
68 ,
69 ,
70 ,
71 ,
72 ,
Download PDF |
|
|
|
|
|
|
|
|
|