|
Tips & Tricks |
|
1. Send data from database in PDF file as
servlet response:
This example retrieves data from MySQL and
sends response to the web browser in the form
of a PDF document using Servlet. This program
uses iText, which is a java library containing
classes to generate documents in PDF, XML,
HTML, and RTF. For this you need to place
iText.jar file to lib folder of your JDK and set
classpath for it. You can download this jar file
from the link http:// www.lowagie.com/iText/
download.html The program below will embed
data retrieved from database in PDF document.
ShowPdf.java import java.io.*;
import java.util.*;
import java.sql.*;
import javax.servlet.*;
import javax.servlet.http.*;
import com.lowagie.text.*;
import com.lowagie.text.pdf.*;
public class ShowPdf extends HttpServlet{
public void doGet(HttpServletRequest req,
HttpServletResponse res) throws
ServletException, IOException{
res.setContentType(“application/pdf”);
//Create a document-object
Document document = new Document();
try{
Class.forName(“com.mysql.jdbc.Driver”);
Connection con =
DriverManager.getConnection(“jdbc:mysql://
localhost:3306/test”, “root”, “root”);
java.util.List list = new ArrayList();
Statement st = con.createStatement();
ResultSet rs = st.executeQuery(“select *
from employee”);
while(rs.next()){
String id = rs.getString(“empId”);
String add = rs.getString(“empAdd”);
list.add(id+” “+add);
}
// Create a PDF document writer
PdfWriter.getInstance(document,
res.getOutputStream());
document.open();
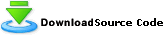
|
|
// Create paragraph
Paragraph paragraph = new
Paragraph(“Data from database:”);
// Add paragraph to the document
document.add(paragraph);
com.lowagie.text.List list1=new
com.lowagie.text.List(true);
Iterator i = list.iterator();
while(i.hasNext()){
list1.add(new
ListItem((String)i.next()));
}
// Add list of data retrieved from
database to the document
document.add(list1);
document.close();
}
catch (Exception e) {
e.printStackTrace();
// Close the document
document.close();
}
}
}
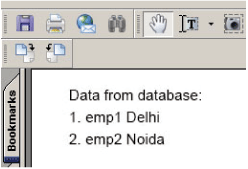
2. Viewing html source of a page running
on the server:
You can view complete html code of a page
running on the server with the help of Java.
This section provides you the complete code of
the above program. This program takes a url |
|
Feb
2008 | Java Jazz Up | 63 |
|
|
View All Topics |
All Pages of this Issue |
Pages:
1,
2,
3,
4,
5,
6,
7,
8,
9,
10,
11,
12,
13,
14,
15,
16,
17,
18,
19,
20,
21,
22,
23,
24,
25,
26,
27,
28,
29,
30,
31,
32,
33,
34,
35,
36,
37,
38,
39,
40,
41,
42,
43,
44,
45,
46,
47,
48,
49,
50,
51,
52,
53 ,
54,
55,
56,
57,
58,
59,
60,
61,
62,
63 ,
64,
65 ,
66 ,
67 ,
68 ,
69 ,
70 ,
71 ,
72 ,
Download PDF |
|
|
|
|
|
|
|
|
|